Custom functions
Note
Custom functions are available only on Enterprise plans.
Note
Make supports the infrastructure and system behavior that takes care of managing and using custom functions. However, our customer care team cannot provide support on how to write and debug your own custom functions.
Functions in Make allow you to transform data. You use functions when mapping data from one module to another. Make offers a variety of built-in functions. On top of that, you can create your own custom functions.
Custom functions allow you to enhance the built-in data transformation capabilities available in the scenario designer.
Custom functions are created using JavaScript.
Create and manage custom functions
You create and manage custom functions in the Functions section, which is accessible from the left menu.
Custom functions belong to a team. All users with the role Team Member can view and use custom functions. To create and edit custom functions, you need to have the Team Admin role.

To create a new custom function:
In the left menu, click Functions.
Click Add a function.
Enter a name and description. The name and description will appear in the list of functions available in the scenario designer.
Important
Requirements for the name:
Must contain only letters and digits.
Cannot start with a digit.
Cannot contain the following words (JavaScript reserved words):
do, if, in, for, let, new, try, var, case, else, enum, eval, null, this, true, void, with, await, break, catch, class, const, false, super, throw, while, yield, delete, export, import, public, return, static, switch, typeof, default, extends, finally, package, private, continue, debugger, function, arguments, interface, protected, implements, instanceof
.
After you save the function in step 4 below, you won't be able to change it.
Click Save.
This creates a function header and an empty function body.
Write the code of the function. Use the keyword
return
to define the return value.See the example functions to get an idea of how to write them.
When done, click Save.
When you save the function for the first time, it immediately becomes available in the scenario designer. Custom functions are marked with an icon to distinguish them from the built-in functions.
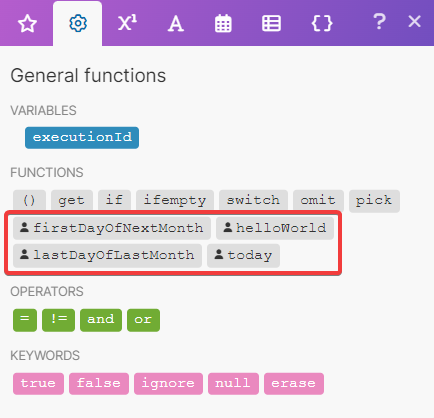
Basic structure of custom functions
The structure of custom functions reflects the structure of regular JavaScript functions. Each function must contain the following:
Function header - the name of the function and the parameters it accepts.
Function body enclosed in curly brackets.
Exactly one
return
statement.
Supported languages
Custom functions support JavaScript ES6. No 3rd party libraries are supported.
The code runs on Make's back end. This means that we do not support functionality that only makes sense in the context of writing browser-executed JavaScript.
Validation of custom functions
Make does not validate the code of your functions. If there are errors in your code, they will appear as errors in the execution of scenarios that use these functions. Invalid functions will cause your scenarios to fail.
Limitations of custom functions
The following limits apply to all custom functions that you create:
A single custom function can not run for more than 300 milliseconds.
The code of a custom function cannot have more than 5000 characters.
Functions must be synchronous. You can't run asynchronous code in your functions.
You can not make HTTP requests from your functions.
You can not call other custom functions from inside of a custom function.
You can not use recursion in your custom functions.
In order to use a custom variable with an iterator, you need to:
Set the value with the custom function in a Set Variable module before the Iterator.
Map the output of Set Variable to the Iterator.
Custom functions version history
Every time you save a custom function, the system creates a new history record. This allows you to compare versions and revert your function to a previous version.
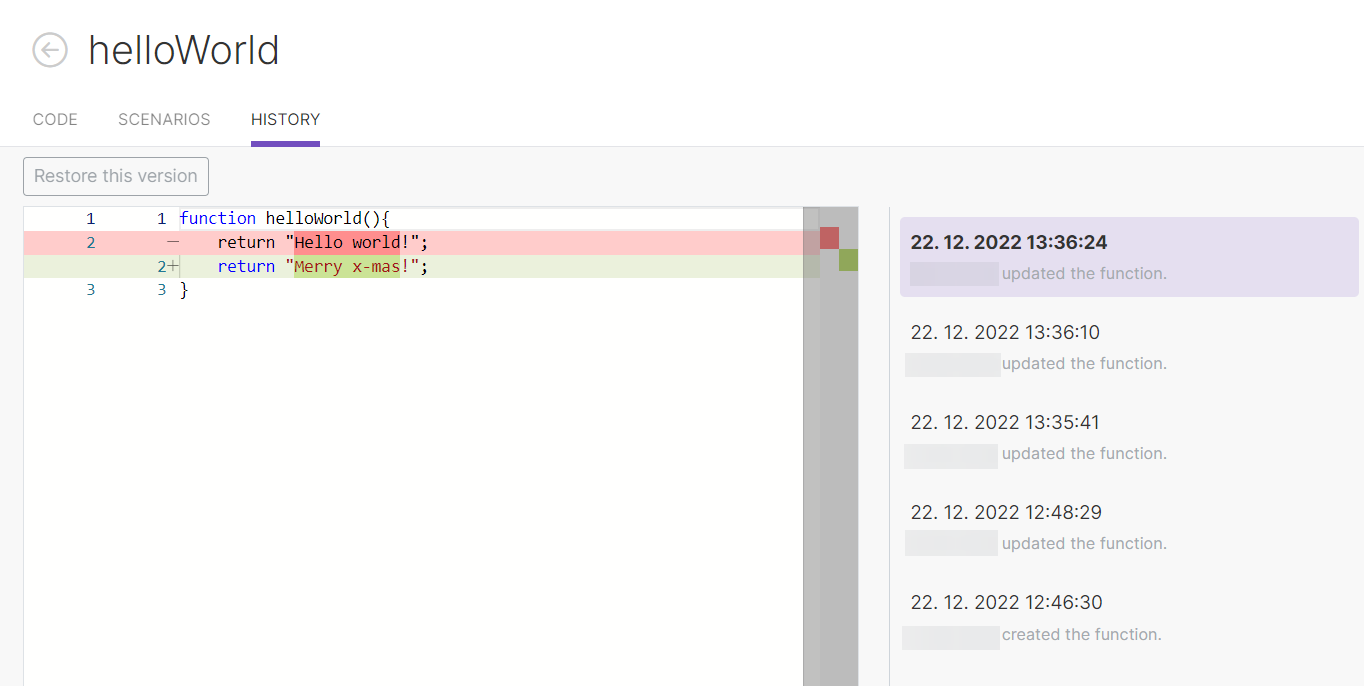
Select a history record to compare the function with the previous version. You can only compare two consecutive versions.
Click Restore this version to revert to the version that you're currently viewing. This creates a new version of the function with the content of the restored version.
Working with dates inside custom functions
Custom functions are executed on Make's servers. Therefore, whenever you work with dates inside a function, the dates will take into account the time zone set for your organization. Read more about how Make handles time zones.
Calling built-in functions from inside of your custom functions
You can use Make's built-in functions in your custom function code.
Built-in functions are available as methods of the iml
object.
The following example uses the Length built-in function.
function useBuiltInFunction(text){ return iml.length(text); }
Debugging custom functions
You can execute your custom function code in the debug console, which is located under the code editor.
Deleting custom functions
You can delete your custom functions in the list in the Functions section.
Before you delete a function, click Edit next to it and then switch to the Scenarios tab. Here, you can check in which scenarios the function is used. When you delete a function that is used in a scenario, the scenario will fail to execute on its next run.
The scenario that uses the deleted function will fail with the following error message:
Failed to map '<field name>': Function '<function name>' not found!
Examples of custom functions
The following functions were written by our developer to help you understand how to write them.
Example: Hello world
Returns the string "Hello world."
function helloWorld() { return "Hello world!"; }
Example: Count the number of working days in a month
Calculates how many working days there are in the month specified by the month's number and a year in the function's arguments. Does not take into account local holidays.
function numberOfWorkingDays(month, year) { let counter = 0; let date = new Date(year, month - 1, 1); const endDate = new Date(date.getFullYear(), date.getMonth() + 1, 0); while (date.getTime() < endDate.getTime()) { const weekDay = date.getDay(); if (weekDay !== 0 && weekDay !== 6) { counter += 1; } date = new Date(date.getFullYear(), date.getMonth(), date.getDate() + 1); } return counter; }
Example: Calculate the number of days between two dates
Calculates the number of days between two dates passed to the function as arguments.
function numberOfDays(start, end) { const startDate = new Date(start); const endDate = new Date(end); return Math.abs((startDate.getTime() - endDate.getTime()) / (1000 * 60 * 60 * 24)); }
Example: Randomization - return a random greeting from an array of greetings
Accepts an array as an argument. Returns a random item from the array.
function randomGreeting(greetings) { const index = Math.floor(Math.random() * greetings.length); return greetings[index]; }